Table of Contents
Understanding Embeds
WordPress allows embedding various types of external content, including posts from other WordPress sites. Using the Embed block, users can integrate content seamlessly by simply pasting a URL. WordPress processes this URL and generates an iframe displaying an optimized embed version.
For example, embedding a WordPress post adds /embed/
to the URL, such as:
- Original URL:
https://example.com/my-post/
- Embed URL:
https://example.com/my-post/embed/
Visiting this embed URL directly in a browser will display a simplified post preview.
How WordPress Handles Embeds
WordPress has supported embeds since version 2.9 using the oEmbed protocol. Key enhancements include:
- WordPress 4.0: Live previews for embeds in the editor.
- WordPress 4.4: WordPress itself became an oEmbed provider.
- WordPress 4.5: Introduced default embed templates.
- WordPress 5.0: Introduced the Embed Block in the Block Editor.
Default WordPress Embed Templates
Important Note:
Site Editor and Block Themes support:
Right now, the Editor doesn’t really support editing embeds.
Even if you’re working with a block theme that uses HTML files for the templates, you still need to add PHP files for custom embed templates.
When embedding a post, WordPress uses built-in templates located in /wp-includes/theme-compat/
:
embed.php
– Base template for post embeds.header-embed.php
– Header template.embed-content.php
– Content template.embed-404.php
– Displays an error if the post is not found.footer-embed.php
– Footer template.
To customize the layout, developers can override these templates by adding them to their theme:
- Place
embed.php
,header-embed.php
, andfooter-embed.php
at the root of the theme. - Place
embed-content.php
andembed-404.php
inside thetemplate-parts/
folder.
Customizing the WordPress Embed Block
Modifying the Embed Styles
To change the appearance of embeds, enqueue a custom stylesheet in functions.php
:
function mytheme_enqueue_embed_styles() {
wp_enqueue_style(
'mytheme-embed-custom',
get_theme_file_uri( 'assets/css/embed-custom.css' ),
[ 'wp-embed-template' ]
);
}
add_action( 'enqueue_embed_scripts', 'mytheme_enqueue_embed_styles' );
Then, define styles in embed-custom.css
:
.wp-embed-featured-image.rectangular {
margin: -25px -25px 25px;
}
.wp-embed-heading {
margin-bottom: 12px;
}
.wp-embed-footer {
margin: 25px -25px -25px;
padding: 25px;
background: #f5f5f5;
}
Alternatively, use inline styles:
function mytheme_embed_inline_styles() {
wp_add_inline_style(
'wp-embed-template',
'.wp-embed-featured-image.rectangular { margin: -20px -20px 20px; }'
);
}
add_action( 'embed_head', 'mytheme_embed_inline_styles' );
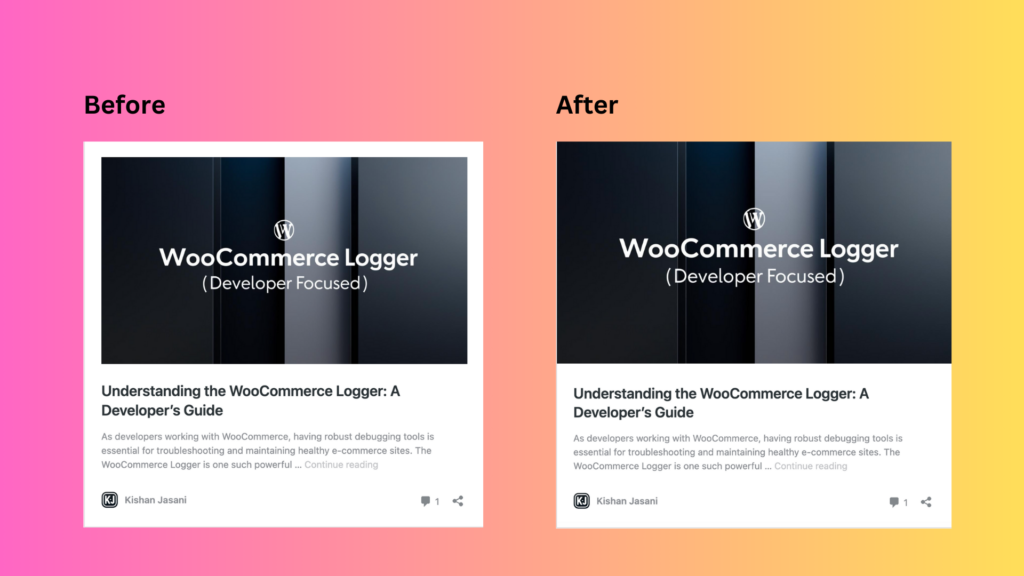
Customizing Embed Images
To enforce a specific image size, register a custom size:
function mytheme_register_embed_image_size() {
add_image_size( 'mytheme-embed-small', 640, 360, true );
}
add_action( 'after_setup_theme', 'mytheme_register_embed_image_size' );
Apply this size to embeds:
function mytheme_set_embed_thumbnail_size() {
return 'mytheme-embed-small';
}
add_filter( 'embed_thumbnail_image_size', 'mytheme_set_embed_thumbnail_size' );
Customizing Embed Excerpts
To modify excerpt length:
function mytheme_embed_excerpt_length( $length ) {
return is_embed() ? 20 : $length;
}
add_filter( 'excerpt_length', 'mytheme_embed_excerpt_length' );
To customize the “Continue reading” text:
function mytheme_embed_excerpt_more( $more ) {
return is_embed() ? 'Read more' : $more;
}
add_filter( 'excerpt_more', 'mytheme_embed_excerpt_more' );
Adjusting Embed Site Title
To display only the site title without the logo:
function mytheme_embed_only_title() {
return sprintf( '<div class="wp-embed-site-title">%s</div>', esc_html( get_bloginfo( 'name' ) ) );
}
add_filter( 'embed_site_title_html', 'mytheme_embed_only_title' );
Adding Metadata (Post Date)
To display the post’s published date:
function mytheme_embed_post_date() {
if ( ! is_single() ) return;
$date = sprintf( '<time datetime="%1$s">%2$s</time>', esc_attr( get_the_date( DATE_W3C ) ), esc_html( get_the_date() ) );
printf( '<p class="wp-embed-post-date">%s</p>', sprintf( __( 'Published on %s', 'mytheme' ), $date ) );
}
add_action( 'embed_content', 'mytheme_embed_post_date' );
Apply styling:
.wp-embed-post-date {
margin-top: 12px;
font-style: italic;
}
Load Template Files from a Plugin
You’re not restricted to storing your embed template files in your theme folder. If you want to reuse a template across multiple projects, you can move it into a plugin and load it from there.
To get started, create a simple plugin with the following file structure:
/plugins/your_plugin_name/
├── index.php
└── templates/
└── embed.php
In the index.php
file, add the necessary plugin header and use the embed_template
filter to change the location of the template:
<?php
/**
* Plugin Name: Kishan Blog Embed Template
*/
function kj_embed_template() {
return plugin_dir_path( __FILE__ ) . 'templates/embed.php';
}
add_filter( 'embed_template', 'kj_embed_template' );
Finally, add your custom embed template code to the templates/embed.php
file.
This is the simplest approach, but it isn’t the most foolproof and doesn’t support post-type–specific files.
For more flexibility, modify the plugin as follows:
function kj_embed_template( $template_path, $type, $template_candidates ) {
foreach ( $template_candidates as $template_candidate ) {
$local_template_path = plugin_dir_path( __FILE__ ) . 'templates/' . $template_candidate;
if ( file_exists( $local_template_path ) ) {
return $local_template_path;
}
}
return $template_path;
}
add_filter( 'embed_template', 'kj_embed_template', 10, 3 );
With this approach, you can also create post-type–specific template files in your plugin, such as /templates/embed-page.php
.
WordPress will check your plugin’s folder first; if no matching file is found there, it will fall back to the theme, and then ultimately to the default files in theme-compat
if needed.
WordPress Embed Hooks
Developers can modify embed behavior using these hooks:
embed_head
– Adds scripts or styles in the embed head.embed_thumbnail_id
– Modifies the featured image ID.embed_thumbnail_image_size
– Changes the thumbnail size.embed_thumbnail_image_shape
– Alters the thumbnail shape.embed_content
– Adds extra content below the embed excerpt.embed_content_meta
– Appends additional metadata.embed_footer
– Inserts scripts before the closing<body>
tag.
Important Notes
- The WordPress embed feature has been available since version 4.4.
- Default embed templates were introduced in version 4.5.
- WordPress sends an
X-WP-embed: true
header in responses, which must be retained when overriding templates. - Ensure that all hooks from default embed templates are included in custom versions to maintain functionality.
Conclusion
Customizing WordPress embeds allows you to create a visually cohesive experience that matches your theme. By tweaking styles, enforcing image consistency, and utilizing hooks, you can enhance the appearance and functionality of embedded content effortlessly.
Related Article:
https://developer.wordpress.org/news/2025/02/customize-wordpress-embeds-to-match-your-theme/
Leave a Reply