The CSS Styling Hierarchy: Understanding How Styles are Applied
Before we dive into the magic of Cascade Layers, let’s break down how styles are traditionally loaded and applied in web browsers. Think of it like a hierarchical system where some styles have more power than others:
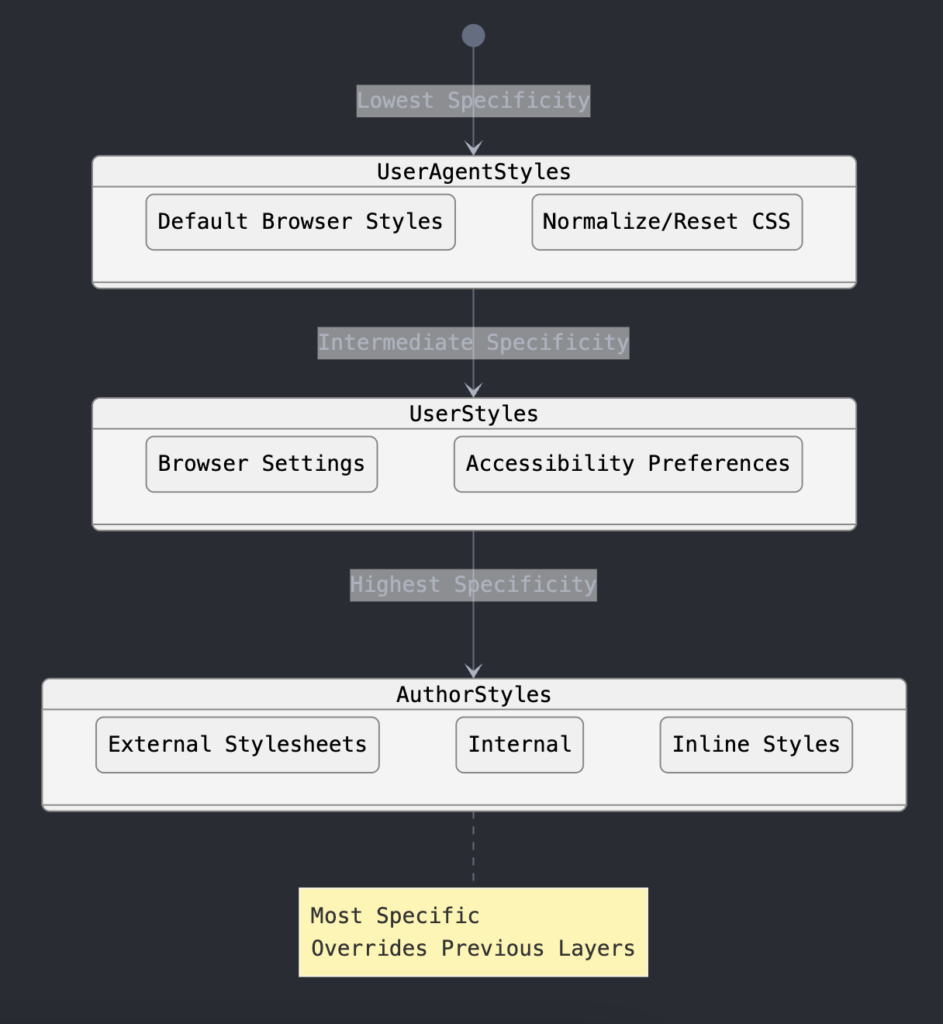
1. User Agent Styles (The Foundation)
Imagine these as the default “factory settings” of your web browser:
- What are they? Basic styles provided by web browsers
- Characteristics:
- Vary slightly between different browsers
- Provide fundamental styling for HTML elements
- Examples:
- Margins and paddings for headings
- Default font sizes
- Basic button appearances
- Link colors
2. User Styles (Personal Preferences)
These are the user’s custom settings:
- What are they? Styles set directly by the website visitor
- Use Cases:
- Accessibility adjustments
- Personal browser settings
- Custom stylesheets users might implement
- Importance: Often overlooked but crucial for inclusive web design
3. Author Styles (Developer’s Domain)
This is where web developers have the most control:
- What are they? Styles written by website developers
- Implementation Methods:
- External stylesheets (most common)
- Internal
<style>
tags - Inline styles directly on HTML elements
- Power: Highest specificity, can override previous layers
The Specificity Puzzle: Challenges Before Cascade Layers
Before Cascade Layers, CSS styling was like a complex puzzle with frustrating limitations:
Common CSS Specificity Nightmares
/* Styles from a third-party library */
button {
background-color: blue;
color: white;
padding: 10px 20px;
}
/* Your project's custom styles */
.theme-button {
background-color: green;
border-radius: 5px;
}
/* Desperate override */
body button {
background-color: red !important;
}
Developers often faced several frustrating challenges when working with CSS. One major issue was the specificity wars, where they constantly struggled to create more specific selectors, leading to increasingly complex CSS just to override existing styles. Another common problem was the overuse of !important
, which acted as a last-resort fix but disrupted the natural cascading behavior of CSS, signaling a breakdown in systematic styling. Additionally, developers had to deal with selector complexity, where deeply nested and hard-to-read selectors made the code less maintainable. All of this resulted in a maintenance nightmare, making styles difficult to understand and increasing the risk of unintended side effects when making changes.
Enter Cascade Layers: A Elegant Solution
Cascade Layers provide a clean, predictable way to manage styles:
/* Explicitly define layers with clear priority */
@layer library, project, override;
@layer library {
button {
background-color: blue;
color: white;
padding: 10px 20px;
}
}
@layer project {
.theme-button {
background-color: green;
border-radius: 5px;
}
}
@layer override {
button {
background-color: red;
}
}
Cascade layers work their magic by providing a clear and structured approach to CSS hierarchy. With explicit layer ordering, developers can define the priority of styles upfront, ensuring a well-organized styling system. This leads to automatic overriding, where styles from lower-priority layers naturally override those from higher ones, eliminating conflicts. As a result, the need for !important
is greatly reduced, allowing for clean and predictable styling without disrupting the natural cascade. Additionally, simple selectors become more effective, maintaining readability and making the CSS easier to manage.
Real-World Design System Example
@layer reset, base, components, utilities;
@layer reset {
/* Normalize styles */
* {
margin: 0;
padding: 0;
box-sizing: border-box;
}
}
@layer base {
body {
font-family: Arial, sans-serif;
line-height: 1.6;
}
}
@layer components {
.button {
padding: 10px 20px;
border-radius: 5px;
}
}
@layer utilities {
.text-center { text-align: center; }
.mt-2 { margin-top: 0.5rem; }
}
This example demonstrates how to use the @layer
rule in CSS with the Cascade Layers feature. This helps control the order of styles, making it easier to manage CSS specificity and overrides.
Breakdown of the Code
@layer reset, base, components, utilities;
- This declares four named layers in a specific order:
reset
,base
,components
, andutilities
. - The order matters! Styles in later layers (
utilities
) will have higher precedence than earlier ones (reset
).
Layer Definitions
Each @layer
block defines styles within a particular layer.
1. Reset Layer (@layer reset
)
@layer reset {
* {
margin: 0;
padding: 0;
box-sizing: border-box;
}
}
This is a CSS reset that removes default browser styles (margin, padding) and sets box-sizing: border-box
to simplify layout calculations.
2. Base Layer (@layer base
)
@layer base {
body {
font-family: Arial, sans-serif;
line-height: 1.6;
}
}
The base styles apply fundamental styles like typography (font-family
, line-height
). Since base
comes after reset
, it applies on top of it.
3. Components Layer (@layer components
)
@layer components {
.button {
padding: 10px 20px;
border-radius: 5px;
}
}
This layer contains reusable components (e.g., .button
). It ensures component styles don’t get overridden by base styles accidentally.
4. Utilities Layer (@layer utilities
)
@layer utilities {
.text-center { text-align: center; }
.mt-2 { margin-top: 0.5rem; }
}
This layer includes utility classes that can be used inline (like Tailwind CSS).
Utilities have the highest precedence in the cascade, meaning they can override component styles.
Why Use @layer?
- Better Control: Helps manage specificity and overrides in a structured way.
- Scalability: Ensures consistent styling when working with multiple contributors or frameworks.
- Overrides Simplified: Utilities (like
.text-center
) can override components (.button
) without needing!important
.
Browser Support
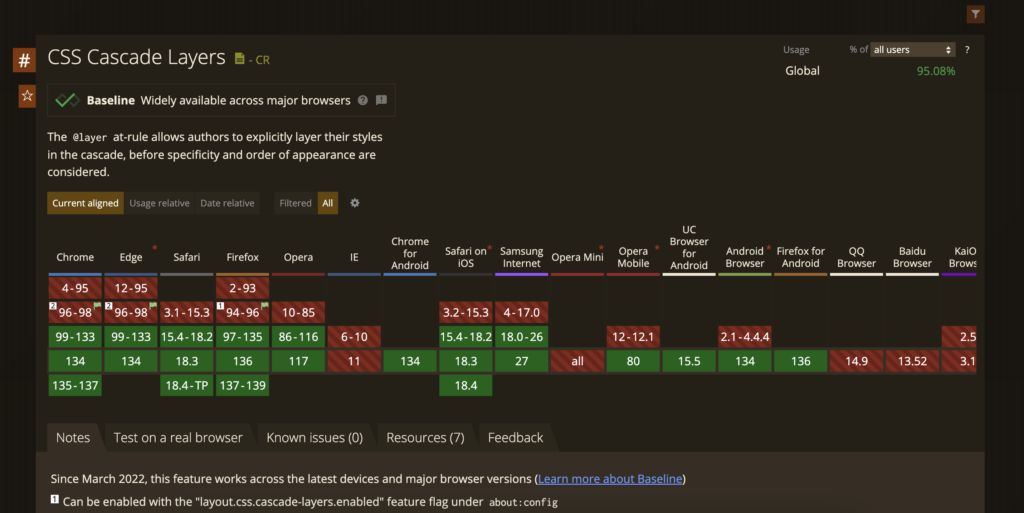
Cascade Layers represent a powerful evolution in CSS. By providing a structured, logical approach to styling, they help developers create more maintainable, readable, and efficient stylesheets.
Leave a Reply