As developers working with WooCommerce, having robust debugging tools is essential for troubleshooting and maintaining healthy e-commerce sites. The WooCommerce Logger is one such powerful tool that often goes underutilized. In this guide, I’ll explore everything you need to know about this feature, from basic usage to advanced customization.
Now that I’ve covered what the WooCommerce Logger is and its basic functionality in the previous blog, let’s dive deeper into how developers can extend and customize the logging system to meet specific needs.
Table of Contents
Accessing WooCommerce Logs
You can access logs through the WordPress admin panel by navigating to: WooCommerce > Status > Logs
From this interface, you can:
- Filter logs by date range, log level, or source
- View log entries directly in the admin panel
- Download logs in CSV format for deeper analysis
For direct server access, logs are stored as .log files in the wp-content/uploads/wc-logs/ directory, which you can access via FTP if needed.
Understanding Log Levels
WooCommerce categorizes logs based on severity, allowing you to filter and prioritize issues:
Log Level | Description |
---|---|
Emergency | System-critical failure, WooCommerce is down |
Alert | Critical error requiring immediate attention |
Critical | Significant issue that needs urgent fixing |
Error | Standard error requiring debugging |
Warning | Potential issue that should be investigated |
Notice | Important but non-critical information |
Info | General runtime messages |
Debug | Detailed logs for development and debugging |
By default, WooCommerce logs events at the Error level or higher, but you can adjust this as needed.
Working with the Logger in Your Code
Here’s how to use the logger in your own code:
$logger = wc_get_logger();
$context = [ 'source' => 'your-plugin-name' ];
Log at different levels:
$logger->debug( 'Detailed information for debugging', $context );
$logger->info( 'General information about a process', $context );
$logger->notice( 'Important information', $context );
$logger->warning( 'Something that needs attention', $context );
$logger->error( 'An error that needs fixing', $context );
$logger->critical( 'A critical issue requiring immediate attention', $context );
$logger->alert( 'A critical issue that should be addressed ASAP', $context );
$logger->emergency( 'System is unusable', $context );
Alternative method:
$logger->log( 'debug', 'Using the log method directly', $context );
The $context
array is important – the ‘source‘ key helps you filter logs by your plugin or theme name.
Configuring Log Handlers
Starting with WooCommerce 2.7, you can configure how logs are stored and processed using log handlers:
1. File Handler (WC_Log_Handler_File)
- The default handler that writes logs to files
- Accessible via WooCommerce Status Logs Files stored in wp-content/uploads/wc-logs/
Example Usage
// Get an instance of WooCommerce logger.
$logger = wc_get_logger();
// Add a log entry using the file handler.
$logger->log( 'info', 'This is a test log entry.', array( 'source' => 'your-plugin-name' ) );
2. Database Handler (WC_Log_Handler_DB
)
- Logs messages into the WordPress database (*_wc_log table).
- Provides a structured UI inside WooCommerce admin.
- Useful for quick debugging without accessing files.
Example Usage
// Get WooCommerce logger.
$logger = wc_get_logger();
// Log an entry into the database.
$logger->log(
'info',
'This is a test database log.',
[
'source' => 'your-plugin-name',
'handler' => 'WC_Log_Handler_DB'
]
);
3. Email Handler (WC_Log_Handler_Email)
- Sends log messages via email.
- Useful for critical errors that require immediate attention.
- Not recommended for frequent debugging (can flood inboxes & impact performance).
Example Usage
// Get WooCommerce logger.
$logger = wc_get_logger();
// Send an email when an error occurs.
$logger->log(
'error',
'A critical issue occurred in the plugin.',
[
'source' => 'your-plugin-name',
'handler' => 'WC_Log_Handler_Email'
]
);
Summary Table
Handler | Storage Location | Use Case | Access Method |
---|---|---|---|
File Handler (WC_Log_Handler_File ) | wp-content/uploads/wc-logs/ | Default handler for logging | WooCommerce โ Status โ Logs |
Database Handler (WC_Log_Handler_DB ) | *_wc_log table in database | Quick debugging in admin UI | WooCommerce โ Status โ Logs |
Email Handler (WC_Log_Handler_Email ) | Sent via email | Immediate alerts for critical issues | Email inbox |
Changing the Default Log Handler
To change the default handler, add this to your wp-config.php
:
define( 'WC_LOG_HANDLER', 'WC_Log_Handler_DB' );
Or
Update the setting in wp-admin:
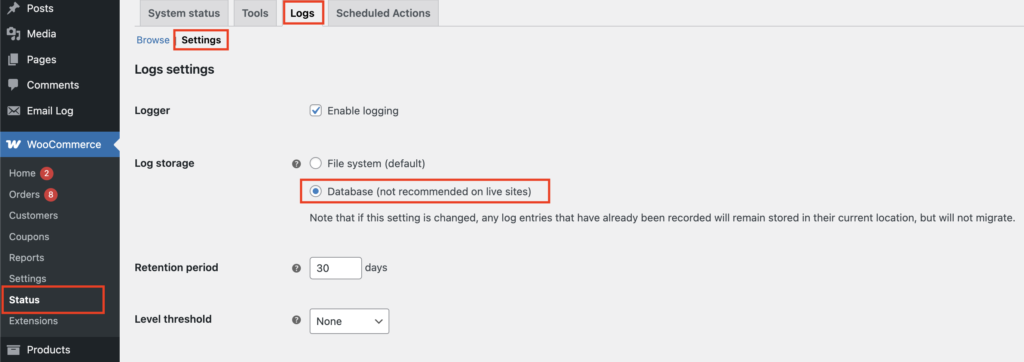
This example sets the database as the primary logging method instead of files.
Creating a Custom Log Handler
For advanced needs, you can create your own custom log handler. There is two ways to create your own custom logger.
1. Use woocommerce_register_log_handlers filter:
function add_custom_log_handler( $handlers ) {
array_push( $handlers, new Custom_Log_Handler() );
return $handlers;
}
add_filter( 'woocommerce_register_log_handlers', 'add_custom_log_handler' );
class Custom_Log_Handler extends WC_Log_Handler {
public function handle( $timestamp, $level, $message, $context ) {
// Implement custom logic.
// e.g: send logs to Slack, AWS, Elasticsearch, etc.
}
}
Or
2. Your custom handler must either implement the WC_Log_Handler_Interface
or extend the WC_Log_Handler
class.
If you need help with this method example, let me know in the comment. Happy to help you! ๐
Best Practices for WooCommerce Logging:
- Use appropriate log levels
- Don’t flood your logs with debug information in production
- Clean up old logs
- Implement rotation to prevent excessive disk usage
- Avoid email handlers for debugging
- They can quickly overwhelm inboxes
- Consider the database handler for easier log reading in the admin
- Implement custom handlers for integration with monitoring services like AWS, Elasticsearch, or Slack
When to Use Logging
Effective logging is particularly useful when:
- Troubleshooting payment gateway issues
- Debugging custom checkout flows
- Monitoring API integrations
- Tracking order processing problems
- Investigating customer-reported issues
By understanding when and how to use the WooCommerce Logger, you can significantly improve your debugging workflow and resolve issues more efficiently.
Conclusion
The WooCommerce Logger is a powerful but often overlooked tool in the developer’s arsenal. By mastering its use, you can gain valuable insights into your store’s operations, quickly identify and fix issues, and create more robust WooCommerce implementations.
Whether you’re using the default logging capabilities or implementing custom handlers for specialized needs, proper logging practices will save you countless hours of debugging and help maintain a healthy e-commerce platform.
Need expert help with WooCommerce development or custom functionality? Hire me for your project.
Leave a Reply